Introduction to MongoDB
When dealing with data, there are two types of data as we know – (i) structured data and (ii) unstructured data. Structured data is usually stored in a tabular form whereas unstructured data is not. To manage huge sets of unstructured data like log or IoT data, a NoSQL database is used.
MongoDB Basic Interview Questions
1. What are some of the advantages of MongoDB?
Some advantages of MongoDB are as follows:
- MongoDB supports field, range-based, string pattern matching type queries. for searching the data in the database
- MongoDB support primary and secondary index on any fields
- MongoDB basically uses JavaScript objects in place of procedures
- MongoDB uses a dynamic database schema
- MongoDB is very easy to scale up or down
- MongoDB has inbuilt support for data partitioning (Sharding).
Some advantages of MongoDB are as follows:
- MongoDB supports field, range-based, string pattern matching type queries. for searching the data in the database
- MongoDB support primary and secondary index on any fields
- MongoDB basically uses JavaScript objects in place of procedures
- MongoDB uses a dynamic database schema
- MongoDB is very easy to scale up or down
- MongoDB has inbuilt support for data partitioning (Sharding).
2. What is a Document in MongoDB?
A Document in MongoDB is an ordered set of keys with associated values. It is represented by a map, hash, or dictionary. In JavaScript, documents are represented as objects:
{"greeting" : "Hello world!"}
Complex documents will contain multiple key/value pairs:
{"greeting" : "Hello world!", "views" : 3}
A Document in MongoDB is an ordered set of keys with associated values. It is represented by a map, hash, or dictionary. In JavaScript, documents are represented as objects:{"greeting" : "Hello world!"}
Complex documents will contain multiple key/value pairs:{"greeting" : "Hello world!", "views" : 3}
3. What is a Collection in MongoDB?
A collection in MongoDB is a group of documents. If a document is the MongoDB analog of a row in a relational database, then a collection can be thought of as the analog to a table.
Documents within a single collection can have any number of different “shapes.”, i.e. collections have dynamic schemas.
For example, both of the following documents could be stored in a single collection:
{"greeting" : "Hello world!", "views": 3}
{"signoff": "Good bye"}
A collection in MongoDB is a group of documents. If a document is the MongoDB analog of a row in a relational database, then a collection can be thought of as the analog to a table.
Documents within a single collection can have any number of different “shapes.”, i.e. collections have dynamic schemas.
For example, both of the following documents could be stored in a single collection:
{"greeting" : "Hello world!", "views": 3}
{"signoff": "Good bye"}
4. What are Databases in MongoDB?
MongoDB groups collections into databases. MongoDB can host several databases, each grouping together collections.
Some reserved database names are as follows:
admin
local
config
5. What is the Mongo Shell?
It is a JavaScript shell that allows interaction with a MongoDB instance from the command line. With that one can perform administrative functions, inspecting an instance, or exploring MongoDB.
To start the shell, run the mongo executable:
$ mongod
$ mongo
MongoDB shell version: 4.2.0
connecting to: test
>
The shell is a full-featured JavaScript interpreter, capable of running arbitrary JavaScript programs. Let’s see how basic math works on this:
> x = 100;
200
> x / 5;
20
6. How does Scale-Out occur in MongoDB?
The document-oriented data model of MongoDB makes it easier to split data across multiple servers. Balancing and loading data across a cluster is done by MongoDB. It then redistributes documents automatically.
The mongos acts as a query router, providing an interface between client applications and the sharded cluster.
Config servers store metadata and configuration settings for the cluster. MongoDB uses the config servers to manage distributed locks. Each sharded cluster must have its own config servers.
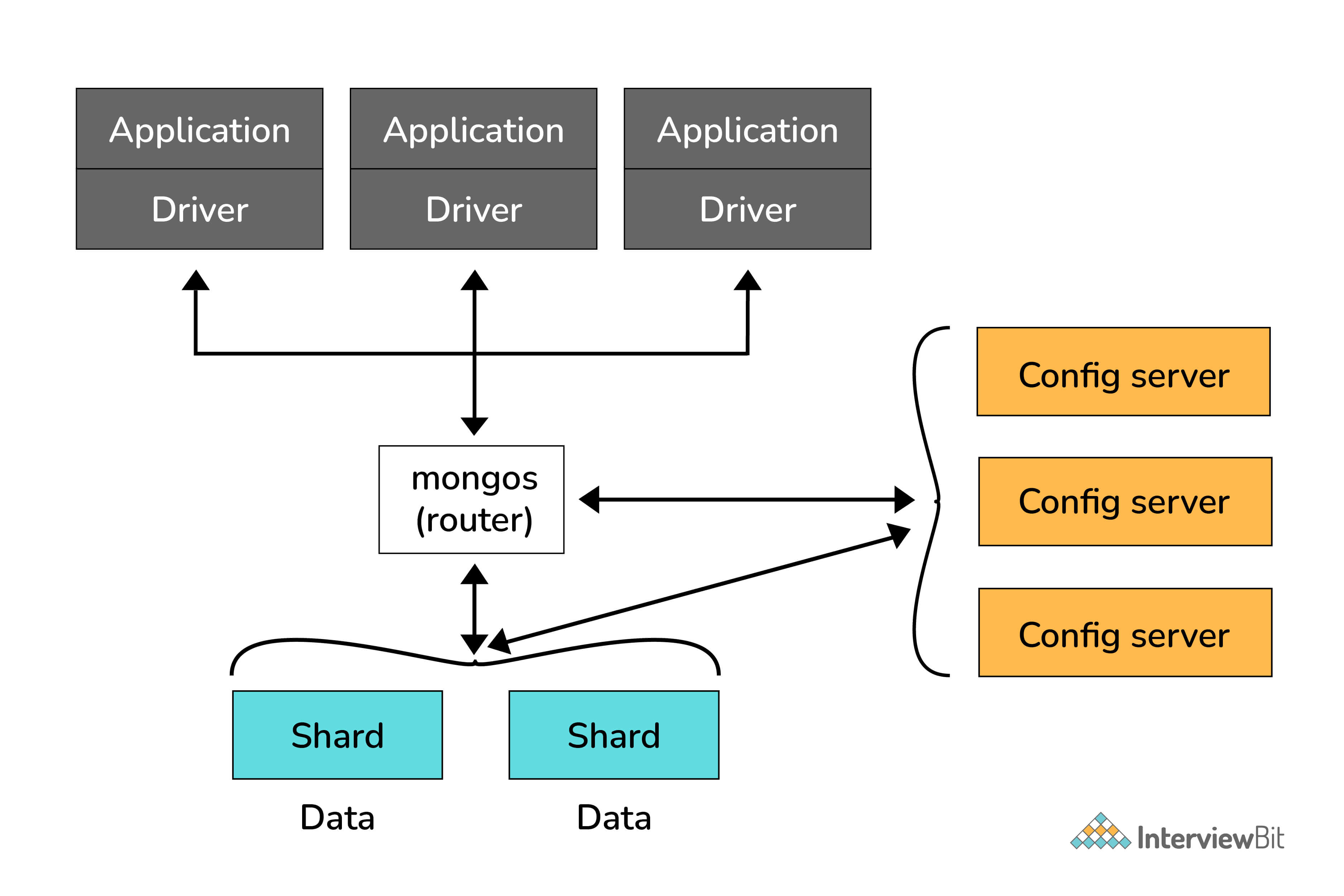
7. What are some features of MongoDB?
- Indexing: It supports generic secondary indexes and provides unique, compound, geospatial, and full-text indexing capabilities as well.
- Aggregation: It provides an aggregation framework based on the concept of data processing pipelines.
- Special collection and index types: It supports time-to-live (TTL) collections for data that should expire at a certain time
- File storage: It supports an easy-to-use protocol for storing large files and file metadata.
- Sharding: Sharding is the process of splitting data up across machines.
8. How to add data in MongoDB?
The basic method for adding data to MongoDB is “inserts”. To insert a single document, use the collection’s insertOne
method:
> db.books.insertOne({"title" : "Start With Why"})
For inserting multiple documents into a collection, we use insertMany
. This method enables passing an array of documents to the database.
9. How do you Update a Document?
Once a document is stored in the database, it can be changed using one of several update methods: updateOne
, updateMany
, and replaceOne. updateOne
and updateMany
each takes a filter document as their first parameter and a modifier document, which describes changes to make, as the second parameter. replaceOne
also takes a filter as the first parameter, but as the second parameter replaceOne
expects a document with which it will replace the document matching the filter.
For example, in order to replace a document:
{
"_id" : ObjectId("4b2b9f67a1f631733d917a7a"),
"name" : "alice",
"friends" : 24,
"enemies" : 2
}
10. How do you Delete a Document?
The CRUD API in MongoDB provides deleteOne
and deleteMany
for this purpose. Both of these methods take a filter document as their first parameter. The filter specifies a set of criteria to match against in removing documents.
For example:> db.books.deleteOne({"_id": 3})
No comments:
Post a Comment